pan069
Member
- Joined
- Jun 4, 2019
- Messages
- 49
Hey everyone,
I have a question re. the Porta to Note effect that I am trying to implement in my MOD player.
My MOD player seems to be working correctly, I'm loading the patterns and sample data and I can playback MODs that don't use effects too much and they sound correct. However, once I started implementing the Porta to Note effect [1] I ran into a bit of a snag. The idea behind this effect is pretty simple, e.g. if you're on note F-1 it allows you to slide up to another note, eg. F-2 with a specified increment (step).
However, the problem I am running into is that it never reached the target note. Take eg. the classic MOD Space Debris [2] which almost immediately starts off with a Porta to Note effect. On the first pattern (played), in channel #3, row 0 (1st) it sets the note to F-1 and triggers a Volume Slide effect (0xA) to volume 10. So far all good, the channel plays at F-1. It then gets to row 12 (13th) when it does a "F-2 .. 308", meaning, "3" (the porta to note effect), it wants to slide to note F-2 (the channel is still on F-1) with steps of 08. So, we don't change the channel frequency, we stay on F-1 but are going to slide to F-2 with steps of 8.
Now, in the Amiga period table the value for F-1 is 640 and the value for F-2 is 320. Meaning there is a 320 difference going from 640 to 320. If we're doing this with steps of 8 then we need 40 ticks to accomplish the fulll slide (320 / 8 = 40). However, there are only 25 ticks available. You see, the Porta to Note effect starts on row 12 (13th) and ends on row 17 (18th) because on row 17 for that channel a Volume Slide effect is started with stops the Porta to Note effect. So, this gives us 5 rows to perform the Porta to Note. The speed is set to 6, so 6 ticks per row. However, tick 0 doesn't do anything for Porta to Note, i.e. Porta to Note is not updated on tick 0, which leavs us with 5 ticks per row in which we can update the Porta to Note. I.e. 5 rows * 5 ticks = 25 ticks. This is not enough, just over halfway there.
So, I am not entirely sure what I am doing wrong. There is clearly something I am overlooking and any thoughts and or insights would be appreicated.
I have added a visual reference that might be helpful to visualise the problem. This screenshot was taken from MiklyTracker (Ubuntu). This tracker does something funky with the representation of the notes (i.e. it shows period 640 as F-4 where in the Amiga period table it is F-1), but that is just a representation thing, it still sounds the same.
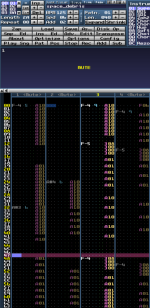
[1] https://github.com/vlohacks/misc/blob/master/modplay/docs/FMODDOC.TXT#L1608
[2] https://markuskaarlonen.com/space-debris
I have a question re. the Porta to Note effect that I am trying to implement in my MOD player.
My MOD player seems to be working correctly, I'm loading the patterns and sample data and I can playback MODs that don't use effects too much and they sound correct. However, once I started implementing the Porta to Note effect [1] I ran into a bit of a snag. The idea behind this effect is pretty simple, e.g. if you're on note F-1 it allows you to slide up to another note, eg. F-2 with a specified increment (step).
However, the problem I am running into is that it never reached the target note. Take eg. the classic MOD Space Debris [2] which almost immediately starts off with a Porta to Note effect. On the first pattern (played), in channel #3, row 0 (1st) it sets the note to F-1 and triggers a Volume Slide effect (0xA) to volume 10. So far all good, the channel plays at F-1. It then gets to row 12 (13th) when it does a "F-2 .. 308", meaning, "3" (the porta to note effect), it wants to slide to note F-2 (the channel is still on F-1) with steps of 08. So, we don't change the channel frequency, we stay on F-1 but are going to slide to F-2 with steps of 8.
Now, in the Amiga period table the value for F-1 is 640 and the value for F-2 is 320. Meaning there is a 320 difference going from 640 to 320. If we're doing this with steps of 8 then we need 40 ticks to accomplish the fulll slide (320 / 8 = 40). However, there are only 25 ticks available. You see, the Porta to Note effect starts on row 12 (13th) and ends on row 17 (18th) because on row 17 for that channel a Volume Slide effect is started with stops the Porta to Note effect. So, this gives us 5 rows to perform the Porta to Note. The speed is set to 6, so 6 ticks per row. However, tick 0 doesn't do anything for Porta to Note, i.e. Porta to Note is not updated on tick 0, which leavs us with 5 ticks per row in which we can update the Porta to Note. I.e. 5 rows * 5 ticks = 25 ticks. This is not enough, just over halfway there.
So, I am not entirely sure what I am doing wrong. There is clearly something I am overlooking and any thoughts and or insights would be appreicated.
I have added a visual reference that might be helpful to visualise the problem. This screenshot was taken from MiklyTracker (Ubuntu). This tracker does something funky with the representation of the notes (i.e. it shows period 640 as F-4 where in the Amiga period table it is F-1), but that is just a representation thing, it still sounds the same.
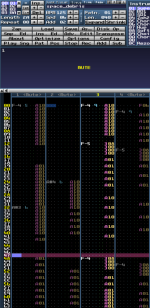
[1] https://github.com/vlohacks/misc/blob/master/modplay/docs/FMODDOC.TXT#L1608
[2] https://markuskaarlonen.com/space-debris