reijo
New Member
- Joined
- Oct 27, 2022
- Messages
- 4
I'm not experienced with 16-bit realmode programming, so I'm sorry if this is an obvious problem. I'm not sure if I've hit some bug, or this is normal.
Anyway, the main question is: is it possible to mix malloc()+free() and _fmalloc()+_ffree() calls in one application? So e.g. normally I use malloc()+free(), but for larger data blocks and selected far pointers I'd use _fmalloc()+_ffree()?
I'm not sure because of this behavior of OpenWatcom's 16-bit compiler (compilation using simply "wcl test.cpp"):
That displays:
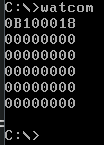
So, every malloc() call I'm doing after _fmalloc() seems to fail. But when I do a malloc() first, THEN _fmalloc(), then everything seems to be fine:
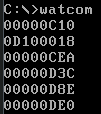
So I'm wondering, if this is some bug, undefined behavior, a logic consequence of something I'm not aware of? I've actually checked the same code with Borland C++ v3.1, and using default settings ("bcc test.cpp") it seems that there's no such problem there -- allocation via malloc() always succeeds (for such small test program at least).
(Also, thread topic fix: it should be "DOS", not "MS-DOS")
Anyway, the main question is: is it possible to mix malloc()+free() and _fmalloc()+_ffree() calls in one application? So e.g. normally I use malloc()+free(), but for larger data blocks and selected far pointers I'd use _fmalloc()+_ffree()?
I'm not sure because of this behavior of OpenWatcom's 16-bit compiler (compilation using simply "wcl test.cpp"):
Code:
#include <malloc.h>
#include <stdio.h>
int main() {
printf("%08lX\n", _fmalloc(2000));
printf("%08lX\n", malloc(0x50));
printf("%08lX\n", malloc(0x50));
printf("%08lX\n", malloc(0x50));
printf("%08lX\n", malloc(0x50));
printf("%08lX\n", malloc(0x50));
return 0;
}
That displays:
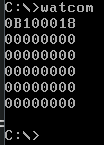
So, every malloc() call I'm doing after _fmalloc() seems to fail. But when I do a malloc() first, THEN _fmalloc(), then everything seems to be fine:
Code:
#include <malloc.h>
#include <stdio.h>
int main() {
printf("%08lX\n", malloc(0x50));
printf("%08lX\n", _fmalloc(2000));
printf("%08lX\n", malloc(0x50));
printf("%08lX\n", malloc(0x50));
printf("%08lX\n", malloc(0x50));
printf("%08lX\n", malloc(0x50));
return 0;
}
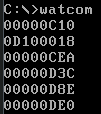
So I'm wondering, if this is some bug, undefined behavior, a logic consequence of something I'm not aware of? I've actually checked the same code with Borland C++ v3.1, and using default settings ("bcc test.cpp") it seems that there's no such problem there -- allocation via malloc() always succeeds (for such small test program at least).
(Also, thread topic fix: it should be "DOS", not "MS-DOS")
Last edited: